React JS, developed by Facebook, is a powerful JavaScript library used for building dynamic and interactive user interfaces, particularly for single-page applications. It allows developers to create large web applications that can update and render efficiently without reloading the page.
Key to React’s popularity is its component-based architecture, enabling developers to build encapsulated components managing their state, then compose them to create complex UIs. Unlike other frameworks, React focuses solely on the view layer, offering flexibility in integrating with other libraries or frameworks for added functionalities.
React’s efficiency stems from its virtual DOM system, which optimizes updates to the real DOM, leading to improved performance. This, combined with its JSX syntax, which allows mixing HTML with JavaScript, makes React a go-to solution for developers seeking to build responsive, high-performance web applications. React’s ecosystem, including tools like Redux for state management and React Router for navigation, further solidifies its position as a versatile and comprehensive solution for modern web development.
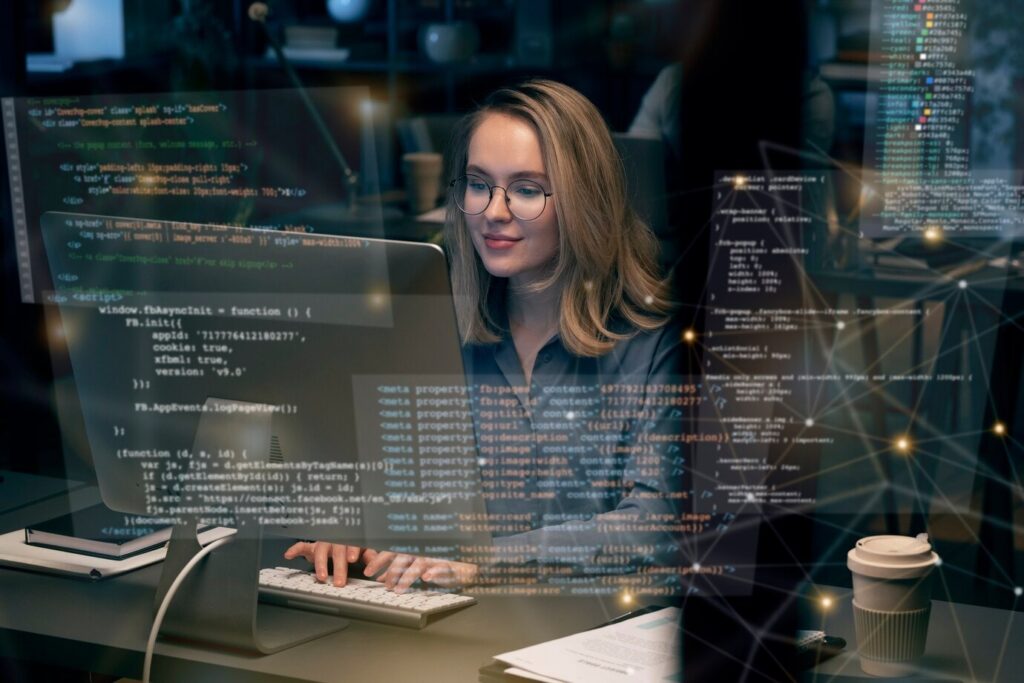
Source: freepik.com
React development services are essential in the modern web development landscape, offering a streamlined approach to building scalable and high-performance web applications. React’s component-based architecture allows for the creation of reusable and maintainable UI components. This modular approach simplifies the development process, making it easier to manage complex projects.
Key aspects of React development services include creating dynamic user interfaces, implementing state management solutions, and integrating with other technologies like Redux for state control, and React Router for navigation. These services often involve developing single-page applications (SPAs) that provide seamless user experiences with fast rendering and efficient data updates.
Moreover, React’s compatibility with various back-end technologies allows for full-stack development capabilities. React development services also focus on optimizing performance, ensuring that applications are not only functional but also responsive and fast.
In summary, React development services encompass a comprehensive suite of solutions tailored to modern web development needs, from building interactive UIs to integrating with various technologies, all aimed at delivering high-quality, efficient web applications.
Core Features of React JS
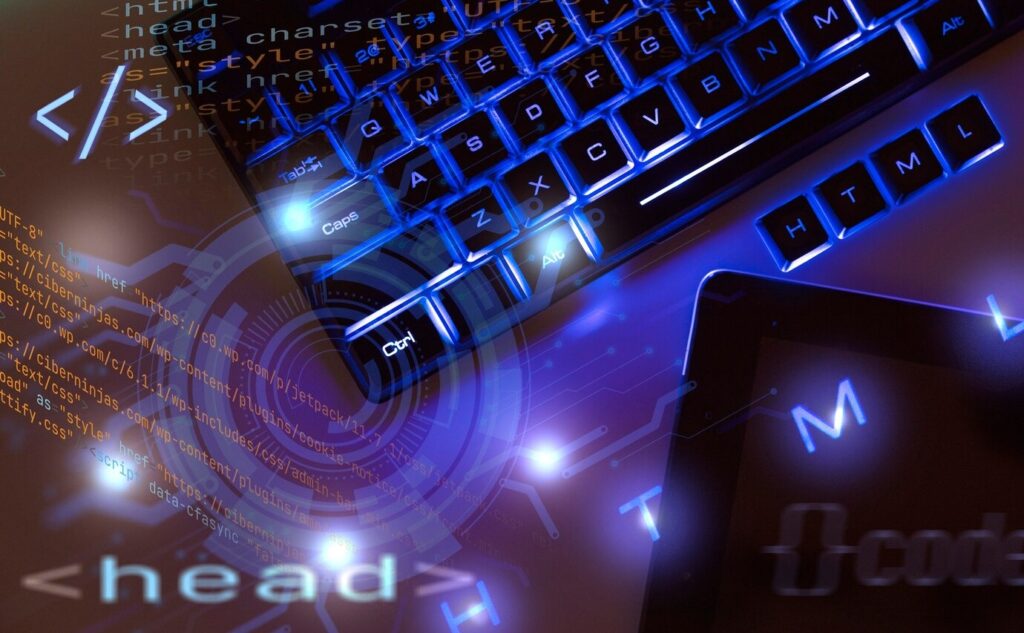
Source: freepik.com
The core features of React JS, which significantly contribute to its popularity and efficiency in web development, include:
- JSX (JavaScript XML): JSX is a syntax extension that allows HTML to be written within JavaScript. This results in a more readable code, especially for building tree structures like DOM.
- Components: React is built around components, which are the heart of any React application. These self-contained, reusable units manage their own state and compose to form complex UIs.
- Virtual DOM: React uses a virtual DOM to optimize DOM manipulation, leading to improved performance. It creates a virtual copy of the UI, which is faster to manipulate than the real DOM.
- One-Way Data Binding: React follows a unidirectional data flow, making it easier to track changes and debug applications.
- State and Props: React’s use of state and props offers a robust way to manage data and render dynamic content.
- Lifecycle Methods: These methods allow execution of code at specific points during a component’s lifecycle, such as creation, updating, and unmounting.
- Hooks: Introduced in React 16.8, Hooks allow for using state and other React features without writing a class.
These features collectively enable developers to build interactive and dynamic web applications efficiently. React’s modular structure not only aids in maintaining and scaling applications but also in streamlining the development process.
Setting Up the Development Environment
Setting up the development environment for React JS involves several key steps to ensure a smooth and efficient workflow:
- Node.js and NPM Installation: The first step is installing Node.js, which includes Node Package Manager (NPM). NPM is essential for managing packages required for React development.
- Creating a React Application: With Node.js and NPM installed, you can use the create-react-app command, a toolchain provided by React to set up a new project. This command sets up the initial project structure and installs the necessary dependencies.
- IDE Setup: Choosing an Integrated Development Environment (IDE) or a code editor is crucial. Popular choices include Visual Studio Code or Sublime Text, known for their React support and user-friendly interfaces.
- Browser Extension: Installing browser extensions like React Developer Tools can significantly aid development. These tools help in debugging and visualizing component hierarchies.
- Version Control: Setting up a version control system like Git helps in managing changes and collaborating with other developers.
- Linting and Formatting Tools: Tools like ESLint and Prettier ensure code quality and consistency.
By following these steps, developers can create an environment that supports the nuances of React development, enabling efficient coding, debugging, and project management.
Building Your First React Application
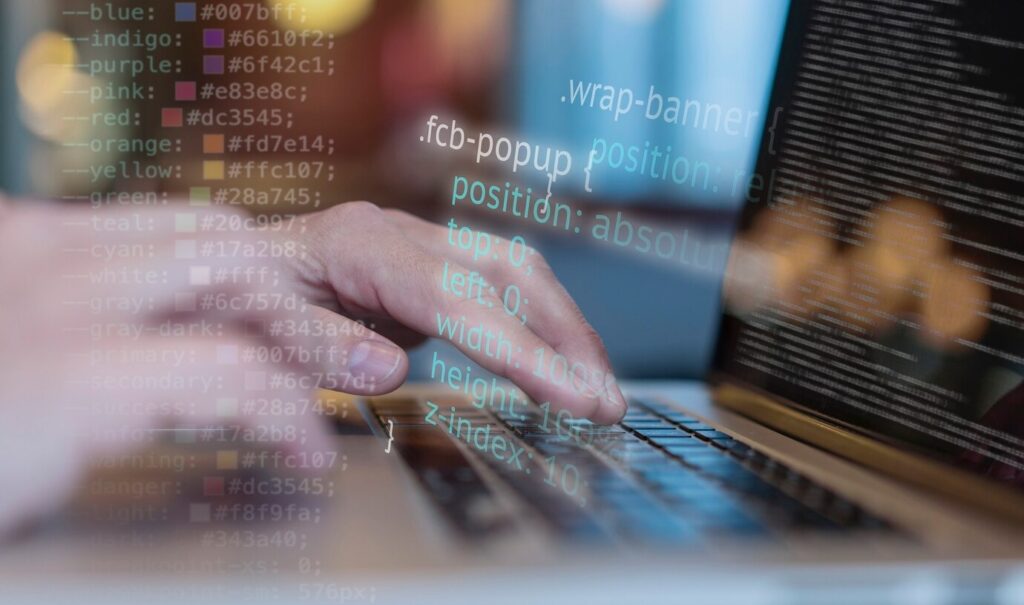
Source: freepik.com
Building your first React application involves a step-by-step process:
- Initialize a New React App: Utilize the create-react-app tool, which sets up the environment and provides a basic template. Run npx create-react-app my-app in your command line, replacing ‘my-app’ with your desired project name.
- Project Structure Understanding: Familiarize yourself with the created project structure. Key directories include ‘public’ for static assets and ‘src’ for React components.
- Developing Components: Start developing React components in the ‘src’ directory. Components are the building blocks of your React app. Create a new component by making a new file ending in .js or .jsx and write your first React component using JSX.
- State and Props Management: Learn how to manage state and props within your components. State allows components to create and manage their own data, while props enable data passing between components.
- Implementing User Interface: Design the UI of your application using JSX, which allows you to write HTML elements in JavaScript code.
- Adding Event Handlers: Incorporate interactivity by adding event handlers to your components. React events, such as onClick and onChange, allow your application to respond to user inputs.
- Running the Application: Use npm start to run your application. This command launches the app in your default web browser.
- Testing and Debugging: Regularly test your application and debug any issues using tools like React Developer Tools in your browser.
By following these steps, you’ll gain hands-on experience in React development, from setting up the project to building and running your first application.
Understanding State and Props
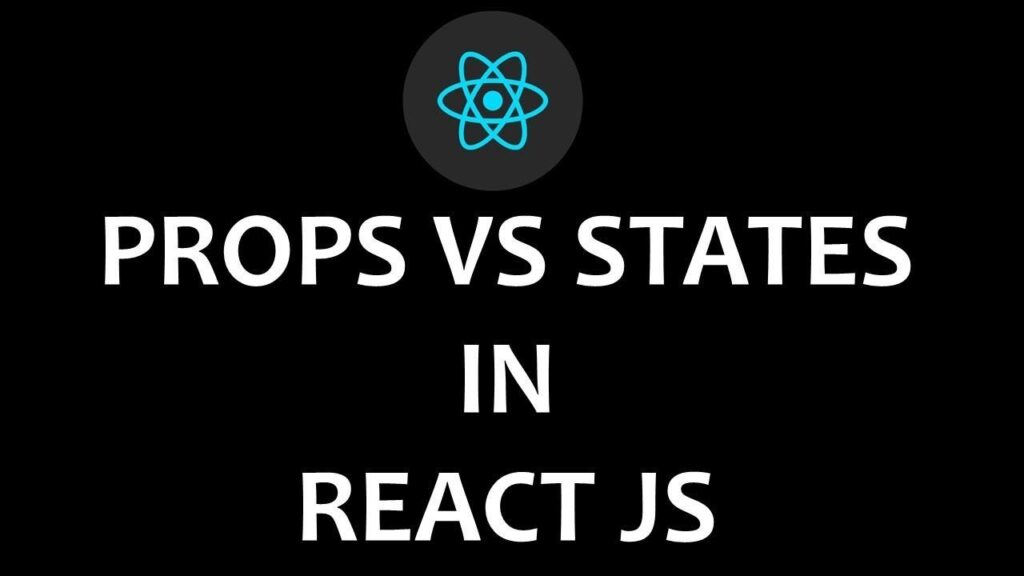
Source: medium.com
Understanding State and Props in React is fundamental to managing data and rendering components effectively.
- State: State represents the component’s data at a given point in time. It’s mutable and local to the component, meaning it can be changed. State is used for data that a component itself can create and modify, such as user input or dynamic UI changes.
- Props (Properties): Props are read-only and immutable, passed from parent to child components. They allow components to communicate with each other and are used to pass data down the component tree. Props maintain the unidirectional data flow in React, making the application easier to debug.
- State vs. Props: Understanding the difference is crucial. State is for data that changes over time, while props are for data that is passed down and remains unchanged by that component.
- Updating State: React provides methods like setState to update the state, which re-renders the component to reflect changes.
- Using Props: Props are accessed in child components and can be used for rendering dynamic data or triggering events in the parent component.
By mastering state and props, developers can create interactive and dynamic React applications, with components that respond appropriately to user interactions and data changes.
Component Lifecycle in React
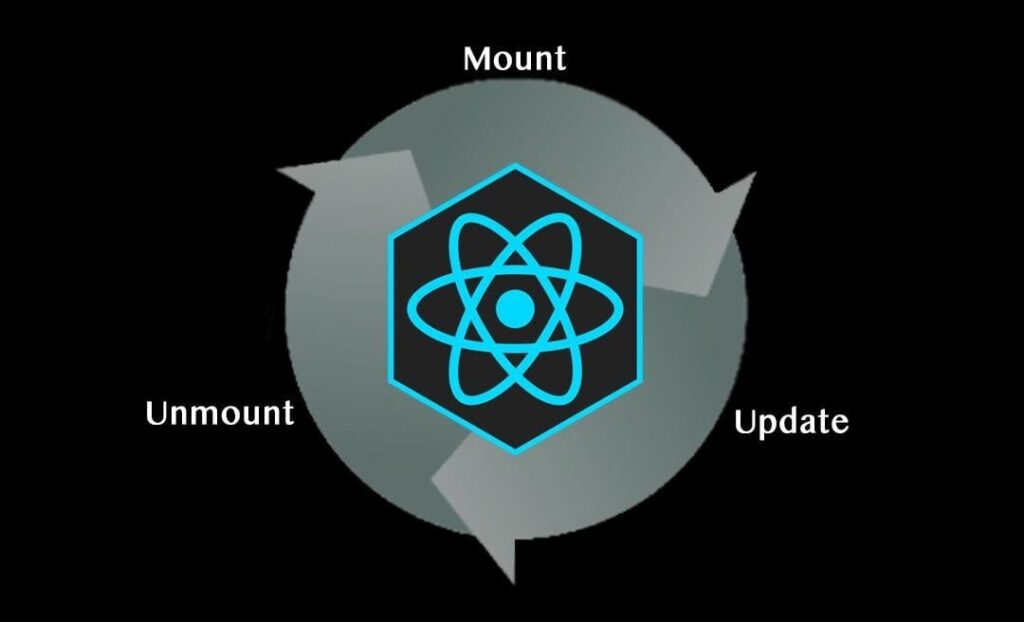
Source: info.keylimeinteractive.com
The Component Lifecycle in React is a series of methods that are automatically called during the lifespan of a component. These lifecycle methods fall into three main phases:
- Mounting: When a component is being created and inserted into the DOM. Key methods include constructor(), render(), and componentDidMount(). componentDidMount() is especially useful for API calls and setting up subscriptions.
- Updating: Occurs when a component’s state or props change. Methods in this phase include render(), shouldComponentUpdate(), getSnapshotBeforeUpdate(), and componentDidUpdate(). These methods are used for performance optimizations and handling component updates.
- Unmounting: The final phase when a component is being removed from the DOM. The key method here is componentWillUnmount(), which is used for cleanup tasks like invalidating timers and canceling network requests.
Understanding these lifecycle methods is essential for managing resources, optimizing performance, and ensuring a component behaves as expected throughout its lifecycle.
Advanced Concepts in React
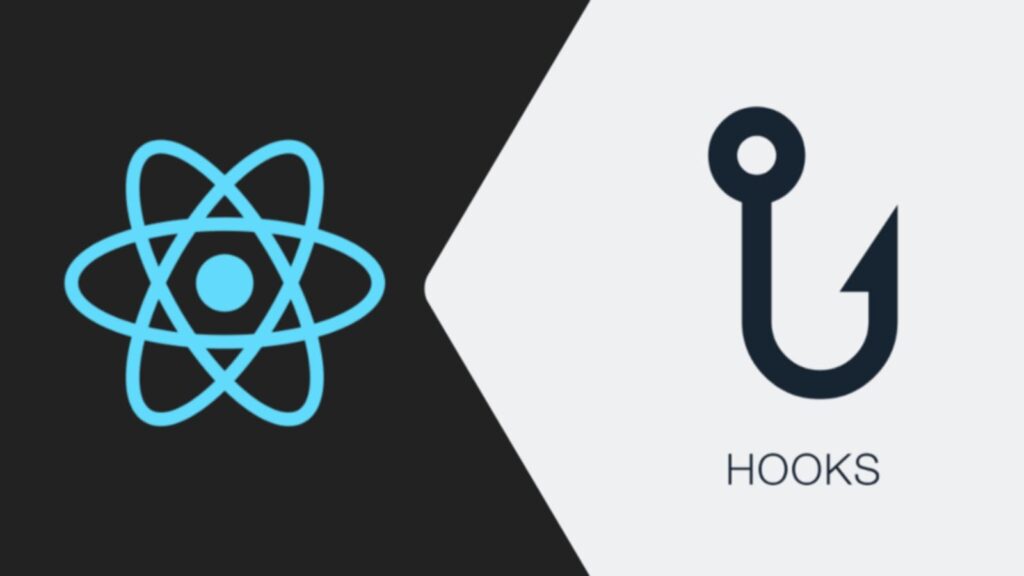
Source: dev.to
enable more complex applications and better state management. Key concepts include:
- Hooks: Hooks like useState, useEffect, and useContext allow functional components to manage state and lifecycle events without classes.
- Context API: This provides a way to share values like configuration data or user info across the entire component tree without explicitly passing props.
- Redux: A state management library often used with React, Redux maintains the state of the entire application in a single immutable state tree.
- Higher-Order Components (HOCs): HOCs are functions that take a component and return a new component, used for reusing component logic.
- Render Props: This pattern involves using a prop whose value is a function to share code between components.
- Fragments and Portals: Fragments let you group a list of children without adding extra nodes to the DOM, while Portals provide a way to render children into a DOM node outside of the parent component’s hierarchy.
These concepts are integral for building scalable, maintainable, and efficient React applications, especially as application complexity grows.
Testing in React
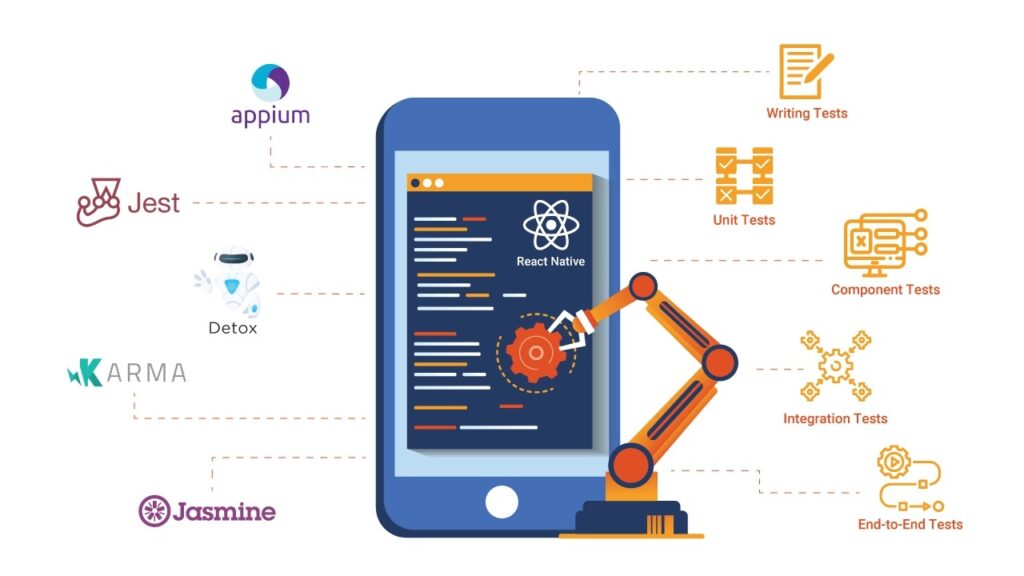
Source: headspin.io
Testing in React is an essential process to ensure the reliability and functionality of the components and the application as a whole. It involves several key aspects:
- Unit Testing: This focuses on testing individual components or functions. Tools like Jest are commonly used, allowing developers to test components in isolation, mock objects, and spy on function calls.
- Integration Testing: This tests the interaction between multiple components. It ensures that combined components work together as expected.
- End-to-End Testing: Tools like Cypress or Selenium are used for end-to-end testing, simulating real user scenarios to test the complete flow of the application.
- Snapshot Testing: A feature of Jest, snapshot testing captures the rendered output of a component and compares it to a reference snapshot to detect changes.
- Testing Hooks and State: Hooks and state changes can be tested using the React Testing Library, ensuring that components respond correctly to user interactions and state updates.
Proper testing in React ensures the development of robust, bug-free applications, enhancing the user experience and application stability.
Best Practices in React Development
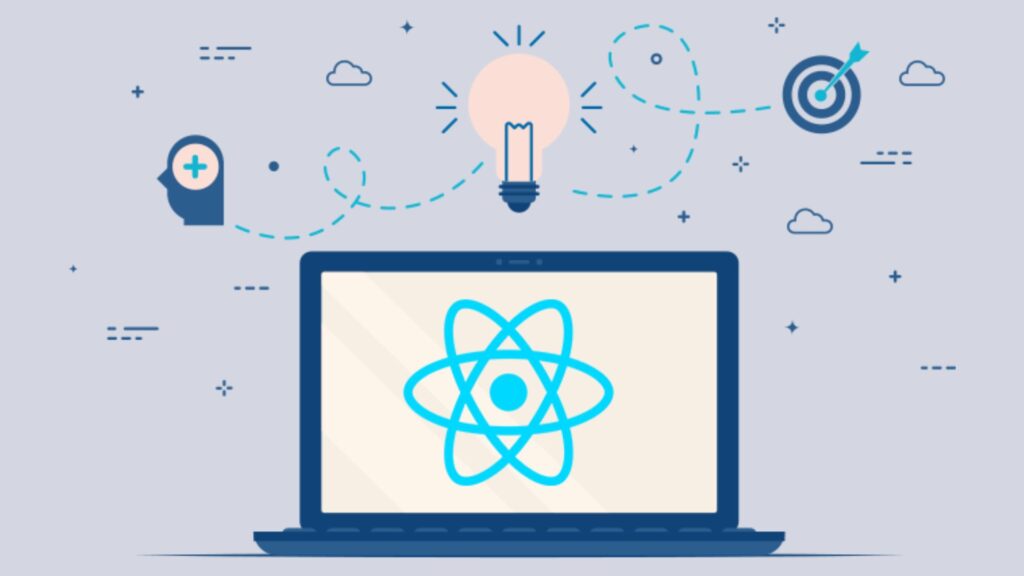
Source: technobrains.io
Adopting best practices in React development is essential for creating efficient, maintainable, and scalable applications:
- Component Reusability: Design components to be reusable across the application. This reduces redundancy and improves maintainability.
- Keeping Components Small and Focused: Create small, single-purpose components for better readability, easier testing, and more straightforward debugging.
- Proper State Management: Efficiently manage state using local state, Context API, or state management libraries like Redux as needed.
- Use of Functional Components and Hooks: Embrace functional components and hooks for cleaner and more concise code.
- Immutable Data Patterns: Treat state as immutable. Use tools like useState and useReducer for state updates.
- Type Checking with PropTypes: Implement PropTypes for type checking, ensuring components are used correctly.
- Efficient Rendering: Optimize rendering performance by preventing unnecessary re-renders and using techniques like memoization.
- Code Splitting and Lazy Loading: Implement code splitting and lazy loading to reduce initial load time and improve performance.
- Unit and Integration Testing: Regularly perform unit and integration tests to ensure code reliability.
- Keeping Up with Latest Practices: Stay updated with the latest React features and updates for continual improvement.
Following these practices helps in building high-quality React applications that are robust, easy to maintain, and performant. In the realm of React development best practices, prioritizing the security of user data is paramount.
In conclusion, React JS stands as a formidable tool in modern web development, offering a robust and flexible framework for building efficient and interactive user interfaces. From its component-based architecture to the efficient handling of state and props, React simplifies the development process, ensuring maintainable and scalable applications.
The integration of advanced concepts like hooks and context API further enhances its capabilities. Adherence to best practices and thorough testing ensures the creation of high-quality, performant applications. As React continues to evolve, staying updated with its latest advancements remains crucial for developers in this dynamic field.